Getting started
What is Rundown Studio?Create an accountRundown
Rundown basicsSettingsRunning a showImport CSV rundownAPICompanion ModuleEvent
Event basicsSharing eventsSharing and outputs
Read-only rundownEditable rundownOutputPDF exportCSV exportAccount
Your teamSubscription and invoicesUpdates
ChangelogAPI
With our v0 API, we have exposed some super handy rundown-specific API calls in order to run your show from your favorite third-party client.
Contents
Getting started
The API uses HTTP GET requests in other to start, pause or advance a rundown. These can be used with tools like Bitfocus’ Companion - where you can use buttons on a Stream Deck to take control of your rundown and other pieces of equipment during a video production.
The API also requires an API token as a security step to ensure only you - or your team mates - have control of any given rundown.
Get the API token
API tokens can be generated from within the Rundown Studio dashboard.
Only team admins can generate and regenerate these tokens, however anyone on your team can read and use the token.
Generating the token can be done in the API section of the dashboard.
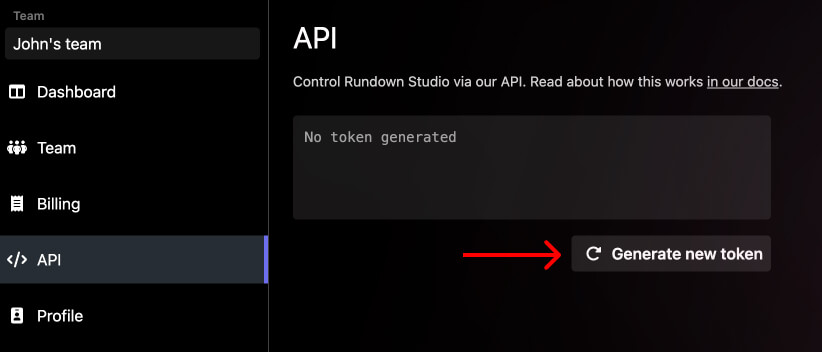
Copy this API token to your clipboard as we will need it a little later.
URL breakdown
An example URL for interacting with our API is as follows…
GET
https://app.rundownstudio.app/api-v0/rundown/<rundown_id>/<start|pause|next>?token=ABCD1234
- app.rundownstudio.app/api-v0/rundown/ ← This is the base URL
- rundown_id ← This is the rundown that you are trying to control
- start|pause|next ← This is the action you want to take
- ?token=ABCD1234 ← This is the token that you have copy/pasted from the dashboard
HTTP API
A detailed breakdown of the available HTTP API endpoints can be found on the swagger documentation page:
Websocket API
The API also provides a websocket endpoint to receive push notifications from our server. It uses the socket.io library which is available in various programming languages:
- JavaScript: socket.io-client on npm
- Python: python-socketio on Read the Docs
- Java: socket.io-client-java on GitHub
- Go: go-socket.io-client on GoDoc
- Rust: rust-socketio on GitHub
The socket.io endpoint receives real-time push messages from the server whenever there is a timing-related change in the rundown. Note that the socket.io endpoint does not listen for any incoming messages.
Enter your API credentials and try it here:
Rundown ID: | |
---|---|
API Token: |
# Click connect ...
Events
The server will send the following events:
serverTime
– The server time sent on a 30s interval, used to sync clocks"2024-01-09T11:45:20.035Z"
rundown
– When the rundown itself changes{
"id": "4LdiPByHcbVZHxjovBQB",
"name": "Example Rundown",
"startTime": "2023-11-23T09:45:00.000Z",
"endTime": "2023-04-01T09:45:00.000Z",
"status": "approved",
"createdAt": "2023-10-23T18:51:22.653Z",
"updatedAt": "2024-01-06T12:55:26.021Z"
}
currentCue
– When the current cue changes{
"id": "ArWdCNok6nS9DjhrQaoD",
"type": "cue",
"title": "Welcome",
"subtitle": "",
"duration": 180000,
"backgroundColor": "#450a0a",
"locked": false,
"createdAt": "2023-10-23T18:51:23.326Z",
"updatedAt": "2023-10-23T18:51:23.326Z"
}
nextCue
– When the next cue changes{
"id": "dkSnrutcDgp5Tq5WGz6P",
"type": "cue",
"title": "Keynote",
"subtitle": "",
"duration": 300000,
"backgroundColor": "#450a0a",
"locked": false,
"createdAt": "2023-10-23T18:51:23.326Z",
"updatedAt": "2023-10-23T18:51:23.326Z"
}
timesnap
– For all timing-related changes like start, stop, next, and previous{
"lastStop": 1704546261727,
"kickoff": 1704546261727,
"running": true,
"cueId": "EF3Rgj4A0IwXV42VP0Ed",
"deadline": 1704547171727
}
Note: The timesnap
event contains timestamps in milliseconds since UNIX epoch. The duration is derived from deadline - kickoff = duration
. The remaining time can be calculated by deadline - now = remaining
.
Code Examples
import { io } from "socket.io-client";
const socket = io('https://socket.rundownstudio.app', {
path: '/api-v0/socket.io',
auth: { rundownId: '[YOUR_RUNDOWN_ID]', apiToken: '[YOUR_API_TOKEN]' },
});
socket.on('connect', () => { console.info('Connection Success') });
socket.on('connect_error', (error) => { console.error('Connection Error:', error); });
socket.onAny((event, payload) => { console.info(event, payload) });
import socketio
sio = socketio.Client()
@sio.event
def connect():
print('Connected to Socket.io')
@sio.on('*')
def catch_all(event, data):
print('Message received', event, data)
auth = { 'rundownId': '[YOUR_RUNDOWN_ID]', 'apiToken': '[YOUR_API_TOKEN]' }
sio.connect('https://socket.rundownstudio.app', {}, auth, None, None, 'api-v0/socket.io')